Java 9 - Stream API improvements
Hi, I am Malathi Boggavarapu working at Volvo Group and i live in Gothenburg, Sweden. I have been working on Java since several years and had vast experience and knowledge across various technologies.
Stream API improvements
Now we learn about Stream API improvements done in java 9.Let's start with the example class Book. It have fields title, authors and price. And we also have methods getBook and getBooks. As their name indicates, getBook method returns a book and getBooks returns list of books.
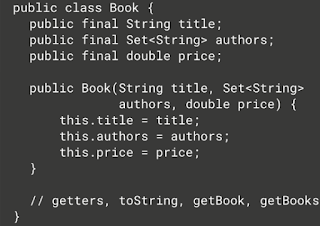
Stream.ofNullable
Now we illustrate Stream.ofNullable method. If we see the below code, when we pass null to the ofNullable method of Stream and call count method on it, it returns zero. And when we call getBook method inside ofNullable method, stream returns the count as one. Try the example!!We are going to look at one more example which is more involved. It illustrates the difference in code before and after java 9 - ofNullable method. We should do extra coding to handle null values. I am not discussing the below code as it is very clear to understand just by looking at it.
takeWhile and dropWhile
We are now going to look at an example which illustrates takeWhile and dropWhile methods. We have an index.html file and this file have a GIT conflict. Git conflicts are normally marked with greater than and less than signs.What we do is, we are going to write a small program that passes any text file containing git conflict and extract conflicting part.So our index.html file looks like below. We are going to extract the highlighted text (Except the HEAD and master lines) using FindGitConflict class
In the above code, we use Files.lines which is a convenient method as it return stream of lines when we pass a filepath as an argument. On the Stream of lines, we use dropWhile method in order to drop all the lines until we find <<<<<<. As soon as we find the line that contains <<<<<<, we need to skip the line by using skip(1) method because we don't want to extarct that line. Next we call takeWhile method and it takes all the lines until it find >>>>>>>> and finally the output is printed to the console.
Try it!!!!
Stream API : New Collectors
Let's revise a bit about the collectors available in java8. In the below example if you see, each element of the Stream is added by 1 and finally collected them to a List using Collectors.toList method.In second example, we use groupingBy method which takes two parameters. The first parameter is predicate and second is toList(). It actually groups even and odd integers and add returns a Map

In java9, we have some advanced collectors. Lets see them with the examples.
Filtering
The first advanced collector is filtering. Here we use a Book as an example which have price, set of authors and a title. Our goal is to take books, a stream of books and find out for each set of authors which books they have written but we have a caveat. We only have to find books whose price is > 10. Only those books should end up in the Set of books for given authors. Try out the example below and check the resultsflatMapping
In this example we see that we take same stream of books and collected into a Map, mapping from a price to all authors that sell a book at this price. Since we are doing grouping on price in this example, ofcourse we are using groupingBy again. The first parameter we are using getPrice. By passing getPrice reference of a book, we are grouping the books by price. However the resulting value of the Map we don't want to have books but we want to have authors. So we still need to do some mapping on the resulting elements in the groups. Now simply mapping the book to get authors won't be enough. Remember getAuthors() return a Set from Book. So what would we end up with is Set of Set of Strings. To flatten the structure we use new flatMapping method. This takes all the streams of authors and flattens them to single stream which will be turned into a Set. And this happens to each group of books group by price.
See the below example of code.
These are fairly advanced usecases for collectors.The additional filtering and flatMapping methods to Collectors even makes java 9 more powerful
So that's all about this course. Hope you enjoyed it. Please post your comments to make the sessions interactive.
Happy Learning!!
Comments
Post a Comment